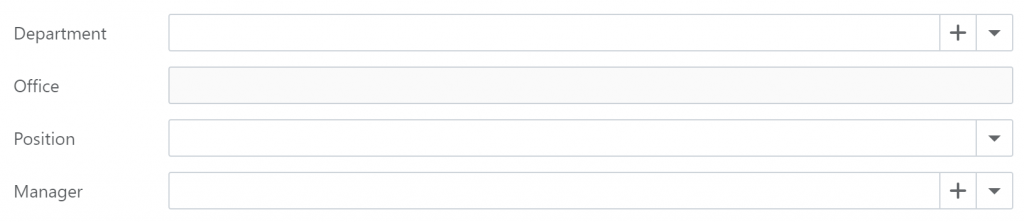
Just a quick post with a few reminders notes about the lookups.
Multicolumn: Already supported
Search: Fields included in the Edit Format
View.CustomizeViewItemControl<LookupPropertyEditor>(this, e => { if (e.Control is DxComboBoxAdapter adapter) { if (e.PropertyName == "contacts") { adapter.ComponentModel.EditFormat = "{0} | {1}"; } //adapter.ComponentModel.CssClass = "test"; }
Search with space in multicolumn:
void OnInput(ChangeEventArgs e) { string value = e.Value.ToString(); var matches = System.Text.RegularExpressions.Regex.Matches(value, @"(\([^)]+?\)|[^(]+)"); if(matches.Count > 1) { Data = dataSource.Where(i => i.Name.ToLower().Contains(matches[0].Value.Trim().Replace("(", "").Replace(")", "").ToLower()) && i.Title.ToLower().Contains(matches[1].Value.Trim().Replace("(", "").Replace(")", "").ToLower())); } else if(matches.Count == 1) { Data = dataSource.Where(i => i.Name.ToLower().Contains(matches[0].Value.Trim().Replace("(", "").Replace(")", "").ToLower())); } else { Data = new List<Employee>(); } }
Hide Actions and Add New ones:
using DevExpress.ExpressApp; using DevExpress.ExpressApp.Blazor.Components.Models; using DevExpress.ExpressApp.Blazor.Editors; using DevExpress.ExpressApp.Blazor.Editors.Adapters; using Xari.Module.BusinessObjects; using Microsoft.AspNetCore.Components.Web; using Microsoft.AspNetCore.Components; using DevExpress.ExpressApp.Blazor.Components.Models.Renderers; using DevExpress.ExpressApp.Editors; using DevExpress.Persistent.BaseImpl; namespace Xari.Blazor.Server.Controllers { public class CustomizePersonInPartyLookupEditorController:ViewController<DetailView> { LookupPropertyEditor currentEditor { get; set; } public CustomizePersonInParteiLookupEditorController() { TargetViewId = "Party_DetailView"; } protected override void OnActivated() { base.OnActivated(); View.CustomizeViewItemControl<LookupPropertyEditor>(this, AddCustomSearchButton, nameof(Partei.Person)); View.CustomizeViewItemControl<LookupPropertyEditor>(this, e => { e.HideNewButton(); e.HideEditButton(); }, nameof(Partei.Art)); } private void AddCustomSearchButton(LookupPropertyEditor obj) { DxEditorButtonModel model = new DxEditorButtonModel(); model.Tooltip = "Search"; model.IconCssClass = "lookupSearchButton"; model.Click = EventCallback.Factory.Create<MouseEventArgs>(this, () => { var os = Application.CreateObjectSpace(typeof(PersonenSuche)); DetailView createdView = Application.CreateDetailView(os, "Person_DetailView", false); createdView.ViewEditMode = ViewEditMode.Edit; Application.ShowViewStrategy.ShowViewInPopupWindow(createdView, () => OnPopupWindowExecute(createdView,obj)); }); var componentModel = ((DxComboBoxAdapter)obj.Control).ComponentModel; var originalButtons = componentModel.Buttons; componentModel.Buttons = builder => { builder.AddContent(0, originalButtons); builder.AddContent(1, model.GetComponentContent()); }; } private void OnPopupWindowExecute(View popupView, LookupPropertyEditor previousEditor) { DetailView dv = (DetailView)popupView; ListPropertyEditor listPropertyEditor = (ListPropertyEditor)dv.FindItem("Person"); if (listPropertyEditor.ListView.SelectedObjects.Count == 1) { previousEditor.PropertyValue = ObjectSpace.GetObject(listPropertyEditor.ListView.CurrentObject); ObjectSpace.CommitChanges(); } } } }
Until next time. XAF out!