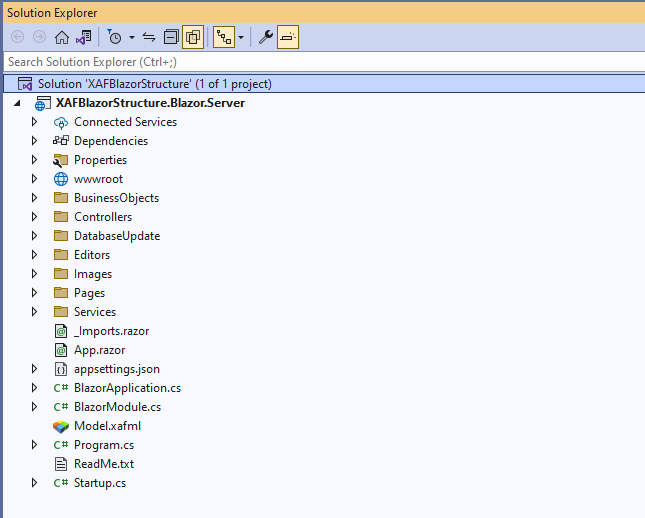
When creating a Blazor application in Visual Studio using XAF, the application is structured into several folders and files, each with a specific purpose. In this article, we will explain each of these folders and files to give you a better understanding of their role in the XAF Blazor application.
While we are focusing on XAF Blazor in this article (as you can have all files in just one project), it is important to note that the concepts discussed also apply to XAF WinForms and WebForms as well. Although the folder and file structure may differ slightly between these different platforms, the underlying concepts remain the same.
For example, the BusinessObjects
folder will still contain your application’s domain classes, regardless of the platform. Similarly, the Controllers
folder will still contain the controllers that handle user requests and execute application logic. The Model.xafml
file will still define the user interface of your application, and the Startup.cs
file will still be responsible for configuring the application’s services and middleware.
Ok, I am getting ahead of myself.
Let’s continue.
Folders
BusinessObjects
The BusinessObjects
folder is where you will store your application’s domain classes, also known as business objects. These classes define the data structure of your application and will be used to create database tables, as well as to represent data in your application.
Controllers
The Controllers
folder contains the controllers that handle user requests and execute application logic. Controllers are responsible for managing the flow of data between the user interface and the application’s business logic.
Editors
The Editors
folder contains custom user interface components that can be used in your application. These editors can be used to customize the appearance of data fields in your application’s user interface.
You can create custom property editors and list editors to provide specific functionality to your application. XAF provides several built-in editors that you can use, but you can also create your own custom editors to meet your specific needs.
Please check our article: Customizing the User Interface in DevExpress ExpressApp Framework (XAF): Main Blocks
Pages
The Pages
folder contains the Razor components that make up the pages of your application. These components are responsible for rendering the user interface and handling user interactions. (Only for Blazor)
Services
The Services
folder contains services that can be used throughout your application. These services can be used to provide functionality such as authentication, data access, and logging.
DatabaseUpdate
The DatabaseUpdate
folder contains scripts that are used to update the database schema of your application. These scripts can be used to add or modify database tables, indexes, or constraints.
The Updater.cs
class inside this folder is responsible for executing these scripts when your application is deployed or when you need to update the database schema manually. See the UpdateDatabaseBeforeUpdateSchema method.
You can use this class to add initial data to your application as XAF already does with the “Admin” and “User” objects of the ApplicationUser entity. See the UpdateDatabaseAfterUpdateSchema method.
wwwroot
The wwwroot
folder is the root directory for static files that will be served by the application. This includes files such as images, CSS stylesheets, and JavaScript files. (Only for Blazor)
Files
App.razor
The App.razor
file is the main component of your application. It is responsible for initializing the application and defining the layout of the user interface. (Only for Blazor)
appsettings.json
The appsettings.json
file contains configuration settings for your application. This includes settings such as connection strings, application-specific settings, and logging settings. (Only for Blazor)
BlazorApplication.cs
The BlazorApplication.cs
file is the entry point for your XAF Blazor application. This file is responsible for configuring the application and starting the web server. (Applies for WinApplication and WebApplication)
The BlazorApplication
class inherits from the AspNetCoreApplication
class, which provides a set of methods and properties that you can use to customize and manage your application.
It is instantiated in the AddXaf
extension method (see the Startup.cs file).
services.AddXaf(Configuration, builder => { builder.UseApplication<XAFBlazorStructureBlazorApplication>();
BlazorModule.cs
The BlazorModule.cs
file defines the modules that make up your application. Modules are used to organize the components of your application and to provide a way to encapsulate functionality. (Applies for WinModule and WebModule)
You can add or remove modules based on your specific requirements. You can also create your own custom modules to provide additional functionality to your application. Each module typically contains its own set of controllers, editors, services, and views.
The Setup
method is a virtual method that is called when the module is registered with the application and is in charge of initializing the newly created XafApplication class instance.
public override void Setup(XafApplication application) { base.Setup(application); //application.CreateCustomModelDifferenceStore += Application_CreateCustomModelDifferenceStore; application.CreateCustomUserModelDifferenceStore += Application_CreateCustomUserModelDifferenceStore; }
Model.xafml
The Model.xafml
file is an XML file that defines the user interface of your application. It defines the layout of the user interface and the data fields that are displayed. (Applies for Model.DesignedDiffs.xafml)
The Model.xafml
file is a critical file in XAF applications. This XML file that describes how the data from your BusinessObjects
will be presented to the user. It also allows us to define Validation Rules, Appearance Rules, Clone Views, Add Filters, Images, Customize DetailView, ListView and a lot more. You can use the XAF Model Editor to modify this file. The Model Editor provides a graphical user interface that allows you to drag and drop fields to create views, layouts, and dashboards.
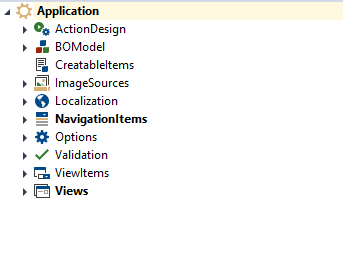
Program.cs
The Program.cs
file contains the Main method, which is the starting point of the application.
The Main methods creates an instance of the web application’s host. The host is responsible for bootstrapping the application and setting up the necessary services and middleware. Then It calls the Run method on the host, which starts the web server and listens for incoming HTTP requests.
host.Run();
Startup.cs
The Startup.cs
file is responsible for configuring the application’s services and middleware. This includes setting up authentication, configuring data access, and defining routing rules.
In the new versions of XAF you can also add XAF Modules like Validation, Conditional Appearance, OAuth authentication, and more.
You can add these modules to your application by calling the AddModule
method in the ConfigureServices
method.
builder.Modules .AddValidation(options => { options.AllowValidationDetailsAccess = false; })
_Imports.razor
The _Imports.razor
file contains a list of namespaces that are used throughout your application. This includes namespaces for common functionality such as the System
namespace and namespaces for your own custom classes. (Only for Blazor)
To summarize, the folders and files in a DevExpress Express App Framework (XAF) application targeting Blazor each have a specific purpose and are essential to the proper functioning of the application. Understanding their roles can help us create effective and efficient applications.
Until next time, XAF out!
#When you get the same questions 3 times you should write an article #NotesToSelf