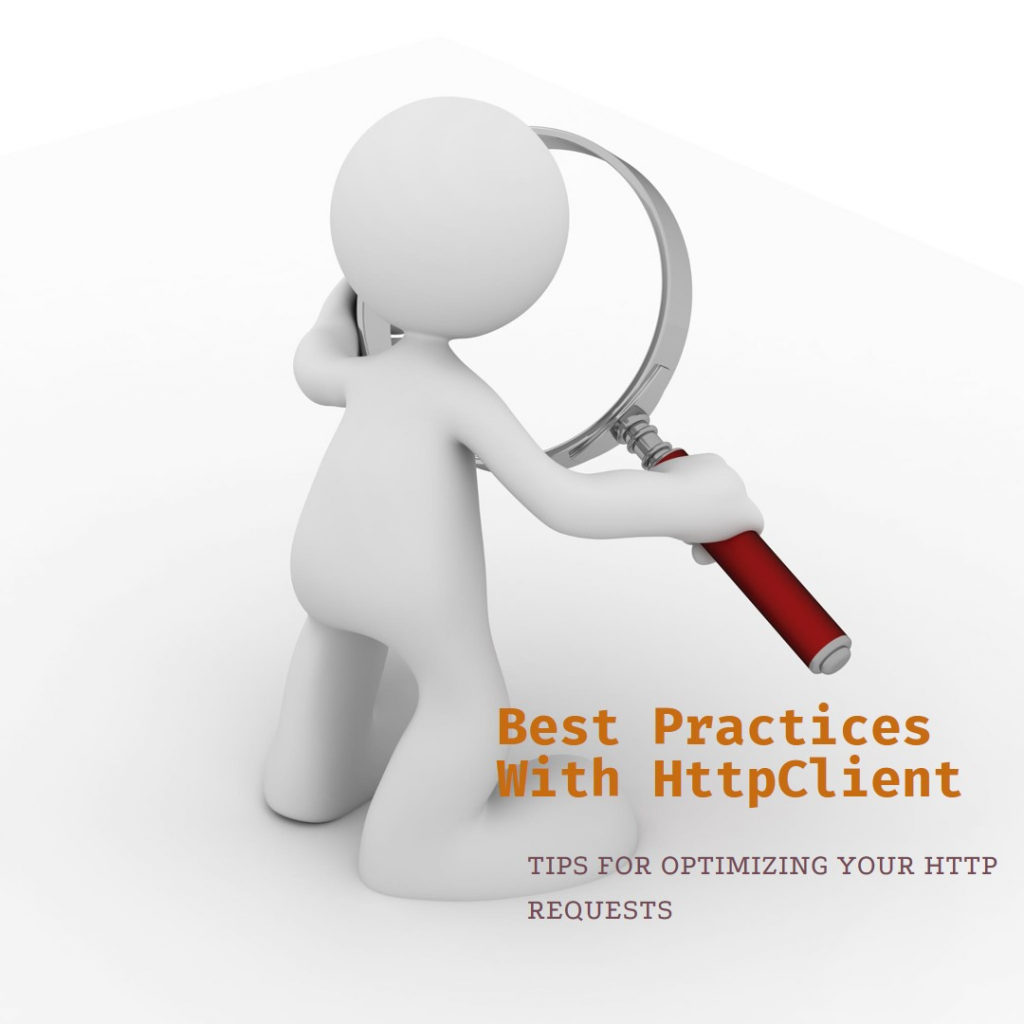
Using HttpClient
in C# effectively is crucial for making HTTP requests and managing HTTP connections in a .NET application. Here are best practices to consider:
1. Reuse HttpClient Instances
- Avoid Creating HttpClient for Each Request: Instantiating
HttpClient
for each request can exhaust the available sockets, leading toSocketException
errors. - Singleton Usage: Use a single instance of
HttpClient
for the lifetime of your application. This can be achieved by using a Singleton pattern, static instance, or dependency injection to manage a long-livedHttpClient
instance.
2. Use HttpClientFactory (ASP.NET Core)
- Leverage HttpClientFactory: In ASP.NET Core applications, use
HttpClientFactory
to manageHttpClient
instances.HttpClientFactory
provides a central location for naming and configuring logicalHttpClient
instances. For example:
services.AddHttpClient("MyClient", client => { client.BaseAddress = new Uri("https://example.com/"); // Set other client settings });
- Benefits: It handles the disposal of HttpClient handlers, provides configurable logging, and allows you to apply policies such as retries with Polly.
3. Configure HttpHandler Appropriately
- Handler Lifetime: When creating a new
HttpClient
instance, you can pass a customHttpMessageHandler
as part of the constructor. Be aware of the handler’s lifetime and how it relates to theHttpClient
‘s lifetime. - Custom HttpHandlers: For advanced scenarios, you might create custom handlers (derived from
HttpMessageHandler
) to intercept and possibly modify requests and responses. For example, adding default headers, logging requests, or handling authentication.
4. Set Timeouts
- Request Timeout: Set appropriate timeout values for your application’s needs. The default timeout is 100 seconds. Adjusting the timeout can prevent your application from hanging indefinitely on requests.
httpClient.Timeout = TimeSpan.FromSeconds(30); // 30 seconds
5. Error Handling
- Try-Catch Blocks: Use try-catch blocks around HTTP requests to handle exceptions, such as
HttpRequestException
. - Check HttpResponseMessage: Always check the
IsSuccessStatusCode
property of theHttpResponseMessage
or useEnsureSuccessStatusCode()
method to ensure the request was successful.
6. Use Polly for Resilience
- Implement Resilience: Use Polly, a .NET resilience and transient-fault-handling library, to implement retries, circuit breakers, and other resilience policies. This is especially useful for applications that communicate with unreliable external services.
7. Security Practices
- HTTPS: Always use HTTPS to ensure the data in transit is encrypted.
- Header Management: Be cautious when setting headers to avoid revealing sensitive information. Remove unnecessary headers.
8. Dispose HttpResponseMessage
- Dispose Responses: Always dispose of the
HttpResponseMessage
instance once you are done processing the response to free up system resources.
9. Use Asynchronous Methods
- Async/Await: Use asynchronous API calls (
HttpClient.GetAsync
,HttpClient.PostAsync
, etc.) to avoid blocking the calling thread. This is particularly important in GUI or ASP.NET applications to keep the application responsive.
10. Testing
- Mocking for Tests: Use interfaces or
HttpMessageHandler
mocks for unit testing your HTTP requests, without actually making live calls.
By adhering to these best practices, you can ensure efficient, reliable, and maintainable HTTP communication in your .NET applications.
Ex: Using HttpHandler
Custom HttpHandler used to create a middleware for logging requests and responses.
public class LoggingHandler : DelegatingHandler { public LoggingHandler(HttpMessageHandler innerHandler) : base(innerHandler) { } protected override async Task<HttpResponseMessage> SendAsync(HttpRequestMessage request, CancellationToken cancellationToken) { // Log request info Console.WriteLine($"Request: {request.Method} {request.RequestUri}"); // Call the inner handler var response = await base.SendAsync(request, cancellationToken); // Log response info Console.WriteLine($"Response: {response.StatusCode}"); return response; } }
Usage with HttpClient
:
var loggingHandler = new LoggingHandler(new HttpClientHandler()); var httpClient = new HttpClient(loggingHandler) { BaseAddress = new Uri("https://example.com") }; // Use httpClient to make requests
For more on HttpHandler take a look at this article by Joche: https://www.jocheojeda.com/2023/07/17/fake-it-until-you-make-it-using-custom-httpclienthandler-to-emulate-a-client-server-architecture/
Ex. Using HttpClientFactory with ASP.NET Core
First, register HttpClientFactory
in Startup.cs
or the program initialization section:
public void ConfigureServices(IServiceCollection services) { services.AddHttpClient(); // Register other services }
Inject and use IHttpClientFactory
in your service or controller:
public class MyService { private readonly IHttpClientFactory _clientFactory; public MyService(IHttpClientFactory clientFactory) { _clientFactory = clientFactory; } public async Task<string> GetExternalApiResponse() { var client = _clientFactory.CreateClient(); var response = await client.GetAsync("https://api.example.com/data"); response.EnsureSuccessStatusCode(); return await response.Content.ReadAsStringAsync(); } }
Integrating Polly with HttpClientFactory
First, add Polly to your project via NuGet:
Install-Package Microsoft.Extensions.Http.Polly
Then, configure HttpClient
with Polly policies in Startup.cs
:
public void ConfigureServices(IServiceCollection services) { services.AddHttpClient("PollyClient", client => { client.BaseAddress = new Uri("https://example.com/"); // Configure other settings }) .AddPolicyHandler(Policy.TimeoutAsync<HttpResponseMessage>(TimeSpan.FromSeconds(10))) // Timeout policy .AddTransientHttpErrorPolicy(p => p.RetryAsync(3)); // Retry policy for transient errors // Register other services }
public void ConfigureServices(IServiceCollection services) { services.AddHttpClient("PollyClient", client => { client.BaseAddress = new Uri("https://example.com/"); // Configure other settings }) .AddPolicyHandler(Policy.TimeoutAsync<HttpResponseMessage>(TimeSpan.FromSeconds(10))) // Timeout policy .AddTransientHttpErrorPolicy(p => p.RetryAsync(3)); // Retry policy for transient errors // Register other services }
Use the named client with policies applied:
public class MyService { private readonly IHttpClientFactory _clientFactory; public MyService(IHttpClientFactory clientFactory) { _clientFactory = clientFactory; } public async Task<string> GetExternalApiResponse() { var client = _clientFactory.CreateClient("PollyClient"); var response = await client.GetAsync("/data"); response.EnsureSuccessStatusCode(); return await response.Content.ReadAsStringAsync(); } }
Http Out!
#When you get the same questions 3 times you should write an article
#NotesToSelf
#Github Issue about RestClient: https://github.com/MelbourneDeveloper/RestClient.Net/issues/75
#Bookmarking : https://www.milanjovanovic.tech/blog/the-right-way-to-use-httpclient-in-dotnet