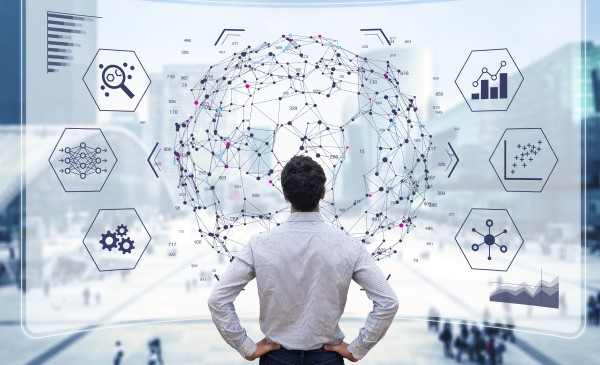
One of the key features of XAF is the XafDataView class, which provides a flexible and efficient way to retrieve and manipulate data in a variety of scenarios.
In this article, we’ll take a closer look at what the XafDataView class is, how it works, and how you can use it in your own projects.
What is the XafDataView class?
The XafDataView class is a powerful data access class that provides a simplified and efficient way to retrieve data from a data store using XPO or EF Core.
We use IObjectSpace.CreateDataView
to create the XafDataView
instance.
The XafDataView class has two specialized subclasses specific to ORM. The IObjectSpace.CreateDataView method determines the current Object Space type and returns either an EFCoreDataView object (for Entity Framework Core) or an XpoDataView object (for XPO) accordingly.
The XafDataView provides a lightweight, read-only list of data records retrieved from a database. Unlike a collection of complete business objects, it is designed to be queried much more quickly, resulting in improved performance.
It does not load complete business objects.
Using the XafDataView class, you can quickly and easily retrieve data from a data store without having to write complex SQL queries or deal with low-level data access APIs. The class provides a wide range of filtering, sorting, and grouping options, making it ideal for working with large datasets.
Let’s see it in action.
Using the XafDataView class is straightforward.
Imagine you have a large database with thousands of customer records, each containing multiple fields such as name, address, and email. Instead of loading all the complete customer objects into memory, which can be memory-intensive and slow, you can use the XafDataView to retrieve only the specific fields you need.
For example, let’s say you only need a simple list of customer names. Using XafDataView, you can create a lightweight data view that only retrieves the customer names from the database, without loading the entire customer objects. This results in faster query execution and reduces memory consumption.
// Create the XafDataView using ObjectSpace.CreateDataView XafDataView dataView = objectSpace.CreateDataView(typeof(Customer), new string[] { "Name" }, null, null); XafDataViewRecord dataRecord = dataView[0];
In this example, we use ObjectSpace.CreateDataView to create the XafDataView instance. We pass the type of Customer, an array of fields we want to retrieve (in this case, just “Name”), and null values for sorting, grouping, and filter parameters.
We then can access a XafDataViewRecord trough its index. The XafDataViewRecord implements IObjectRecord (The interface for business object wrappers that XAF uses in different List View data access modes.)
After assigning the record to the dataRecord
variable, you can access the individual field values using the record’s properties or methods. These properties or methods depend on the structure of the data view and the fields included in it.
For example, if the XafDataView
was created with the fields “Name” and “Email”, you can access the values of those fields from the dataRecord
object like this:
string name = dataRecord["Name"].ToString(); string email = dataRecord["Email"].ToString();
Once you have created the XafDataView instance, you can start filtering and sorting the data using the various methods and properties provided by the class.
For example, you can use the Criteria property to apply a filter to the data:
dataView.Criteria= CriteriaOperator.Parse("[Category] = ? AND [Price] < ?", "Tech", 100000);
This example sets the criteria property to only return objects where the “Category” property equals “Tech” and price is bigger than 100000.
You can also use the Sorting Property to specify the sorted columns within the data view.
Now, let’s see another way to create XafDataViews: DataViewExpression
List<DataViewExpression> dataViewExpressions = new List<DataViewExpression>(); dataViewExpressions.Add( new DataViewExpression("ID", new OperandProperty("ID"))); dataViewExpressions.Add(new DataViewExpression( "Name.UpperCase", new FunctionOperator(FunctionOperatorType.Upper, new OperandProperty("Name")))); dataViewExpressions.Add(new DataViewExpression( "Count", new AggregateOperand("Sales", Aggregate.Count))); var dataView = objectSpace.CreateDataView(typeof(Sale), dataViewExpressions, null, null);
The resulting dataView will contain the specified fields and calculated values based on the expressions provided.
And that’s a wrap for this article!
Remember:
If you are using XAF, the XafDataView is a must-have tool that will help you to easily and efficiently query your data.
Until next time, XAF out!
Note: For the EFCoreDataView I could not find the link to the Docs.
#When you get the same questions 3 times you should write an article
#NotesToSelf