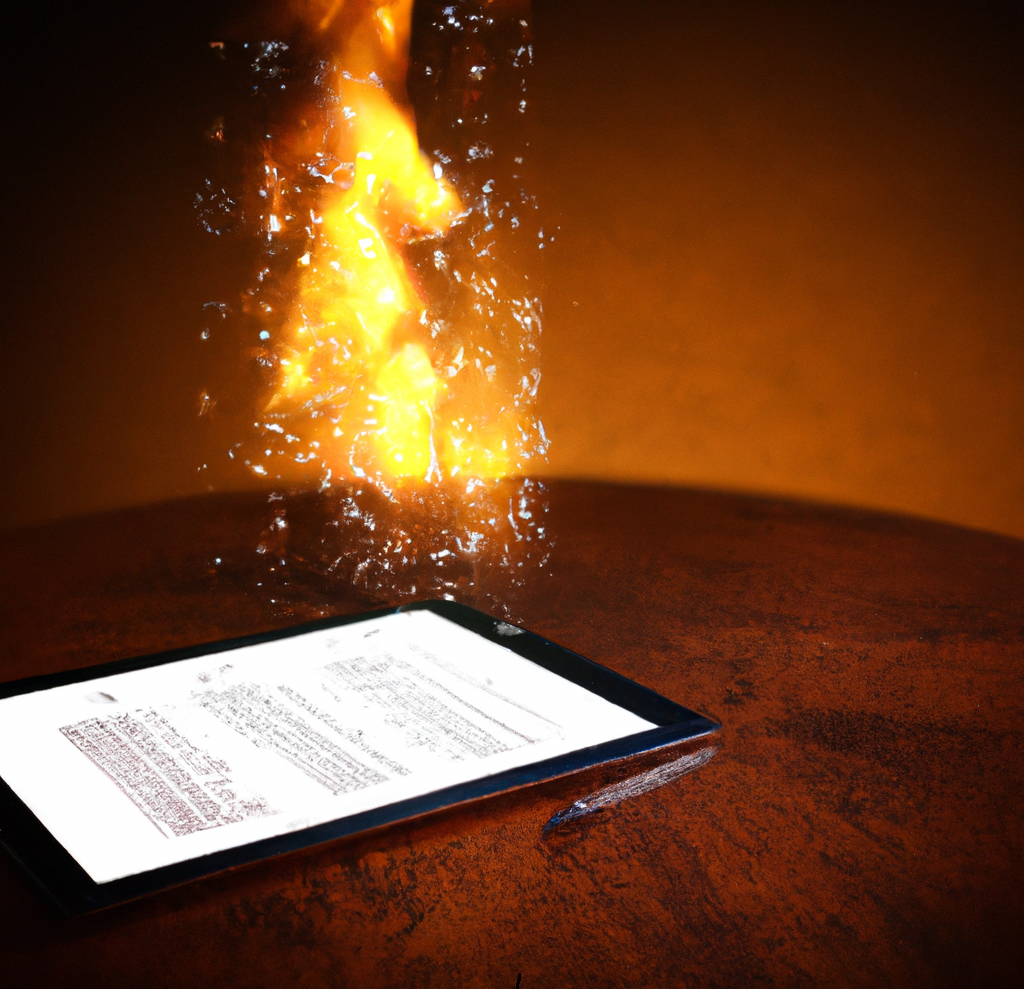
One of the key features of XPO is the XPView class, which provides a flexible and efficient way to work with data from multiple tables or queries.
Let’s dig deeper.
What is the XPView Class?
The XPView class is a lightweight, read-only data source that can be used to display data in a grid or other user interface component. It is particularly useful when loading only partial data or when you need to join data from multiple tables or perform complex queries that cannot be easily expressed using the standard XPO querying API.
When comparing an XPCollection, which is populated with persistent objects, to the XPView, it’s important to note that the XPView serves a different purpose. Unlike an XPCollection, the primary role of the XPView is to facilitate data display. Therefore, when a new instance of the XPView class is created, its XPView.Properties collection remains empty. It is our job to fill that collection.
XPView is slightly faster than XPCollection, because it allows you to eliminate the amount of loaded data.
Unlike XPCollection, XPView does not load objects. So, you cannot obtain an item from XPView and access its properties like in case of XPCollection.
XPView allows you to define various data structures without the necessity to modify the global data structure. It provides flexible capabilities to query only that data that is necessary, join data from multiple tables without loading unnecessary data, and even perform some aggregations using capabilities provided by a SQL database.
Let’s see it in action.
Here is an example of how to create an XPView object and use it to display data in a grid:
using DevExpress.Xpo; XPView view = new XPView(Session.DefaultSession, typeof(Customers)); // Specify the columns to display in the grid view.Properties.AddRange(new ViewProperty[] { new ViewProperty("Name", SortDirection.None, "Name"), new ViewProperty("Address", SortDirection.None, "Address"), new ViewProperty("City", SortDirection.None, "City"), new ViewProperty("State", SortDirection.None, "State"), new ViewProperty("Zip", SortDirection.None, "Zip") }); // Bind the XPView object to a grid control gridControl1.DataSource = view;
In this example, we create an XPView object. If the session isn’t specified, the view works with the default session. We then specify the columns to display in the grid by creating ViewProperty objects and adding them to the XPView object’s Properties collection. Finally, we bind the XPView object to a grid control using the DataSource property.
Using the XPView class, you can perform complex queries that involve joins, grouping, and aggregation. For example, here is an example of how to group data by a particular column and calculate the average value of another column:
XPView view = (Session.DefaultSession, typeof(Orders)); // Group the data by the CustomerName column and calculate the average value of the OrderAmount column view.GroupBy = new string[] { "CustomerName" }; view.Properties.AddRange(new ViewProperty[] { new ViewProperty("CustomerName", SortDirection.None, "CustomerName"), new ViewProperty("AverageAmount", SortDirection.None, CriteriaOperator.Parse("Avg(OrderAmount)"), false) }); // Bind the XPView object to a grid control gridControl1.DataSource = view;
In this code, we group the data by the CustomerName column and calculate the average value of the OrderAmount column using a CriteriaOperator object. We then specify the columns to display in the grid by creating ViewProperty objects and adding them to the XPView object’s Properties collection.
Let’s see another example:
using DevExpress.Xpo.DB; XPView view = new XPView(unitOfWork, typeof(Person)); view.AddProperty("Name", "Concat([FirstName], ' ', [LastName])"); foreach(ViewRecord record in view) { Console.WriteLine(record["Name"]); }
This code retrieves data from the “Person” table, creates a new property called “Name” by concatenating the first and last names, and then iterates over each ViewRecord in the XPView to display the “Name” property value.
Data from multiple tables
SELECT (customer.FirstName + ' ' + customer.LastName) AS "Contact Name", address.City, (SELECT SUM(order.Amount) AS "Total Amount" FROM Orders order WHERE customer.ID = order.CustomerID) as "Total Amount" FROM Customers customer LEFT JOIN Addresses address ON customer.AddressID = address.ID
using DevExpress.Xpo; // ... XPView view = new XPView(unitOfWork, classInfo); view.AddProperty("Contact Name", "Concat([FirstName], ' ', [LastName])"); view.AddProperty("City", "[Address.City]"); view.AddProperty("Total Amount", "[Orders].Sum([Amount])");
And that’s a wrap for this article!
We demonstrated how XPView can be used to manipulate and display data from a specific table and/or multiples tables while providing flexibility in defining custom properties for retrieval and presentation purposes.
Remember:
If you are using DevExpress ORM XPO in your .NET application, the XPViewclass is a must-have tool that will help you to easily and efficiently query your data.
Until next time, XPO out!
#When you get the same questions 3 times you should write an article
#NotesToSelf