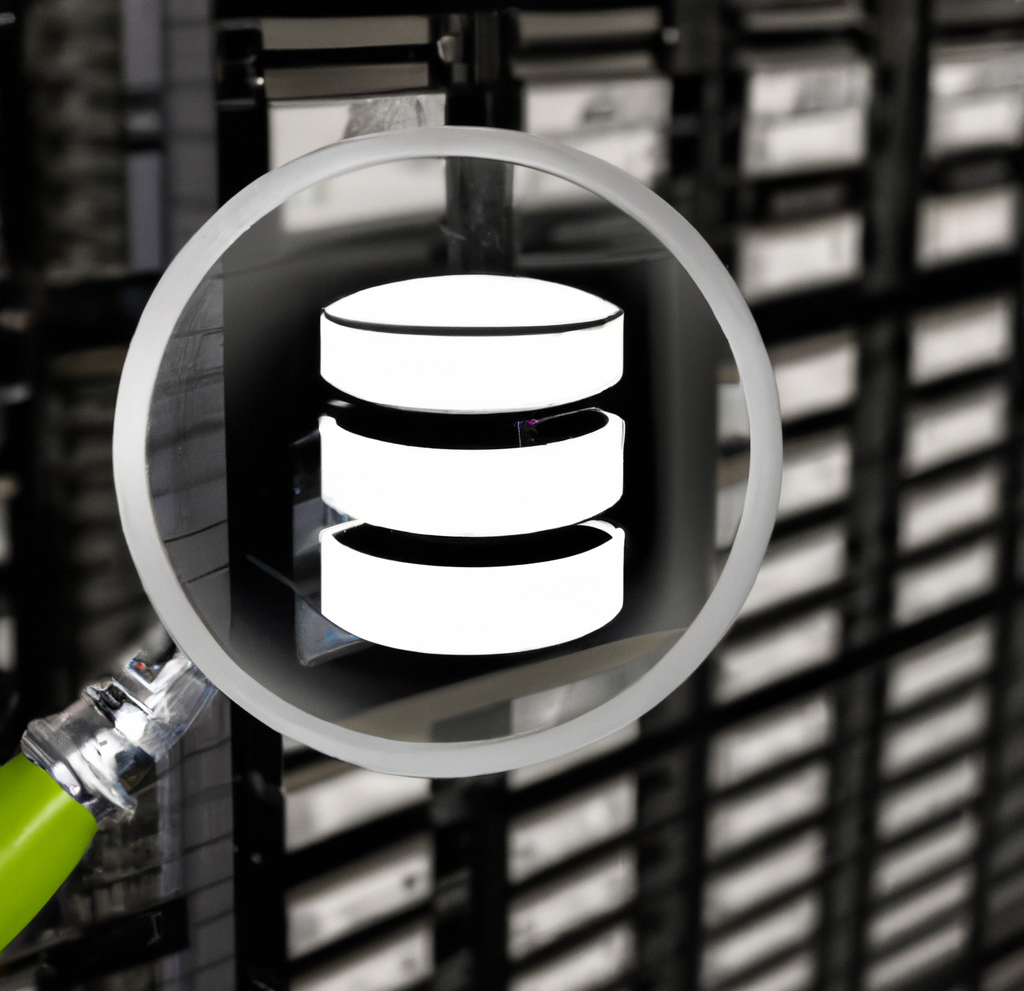
One of the key features of XPO is the XPQuery class, which provides a flexible and efficient way to query data from the database.
Let’s dig deeper.
What is the XPQuery Class?
The XPQuery class provides a powerful and flexible way to query data that is stored in XPO objects, as well as in external data sources. The XPQuery class is designed to work with LINQ, which is a powerful query language that is used to query objects in .NET.
Let’s see it in action.
To use the XPQuery class, you must first create an instance of the class and then define a LINQ query that will be used to query the data. The following code demonstrates how to create an instance of the XPQuery class and define a LINQ query:
using DevExpress.Xpo; using System.Linq; XPQuery<Customer> customersQuery = new XPQuery<Customer>(session); var customers = from customer in customersQuery where customer.City == "London" select customer;
In this example, we create an instance of the XPQuery class that will query the Customer objects that are stored in the database. We then define a LINQ query that will select all the customers who are located in London.
Once we have defined the query, we can then execute it by using the ToList
method:
foreach (var customer in customers.ToList()) { Console.WriteLine(customer.Name); }
This code will iterate over the results of the query and print the names of all the customers who are located in London.
The XPQuery class can be used to query data from any XPO object. Meaning any of the databases supported by XPO. (SQL Server, Oracle, MySQL, etc.)
See below.
using DevExpress.Xpo.DB; string connectionString = "Data Source=(local);Initial Catalog=Xari;Integrated Security=True"; IDataStore dataStore = XpoDefault.GetConnectionProvider(connectionString, AutoCreateOption.None); Session session = new Session(dataStore); XPQuery<Customer> customersQuery = new XPQuery<Customer>(session); var customers = from customer in customersQuery where customer.City == "London" select customer;
This code creates a new Session
instance that is connected to the Xari database and then we use the XPQuery class with the same LINQ query syntax as before.
Anonymous objects to the rescue
Loading partial data using anonymous objects in XPO can be useful when you want to optimize your queries and only load the specific properties that you need from an object, rather than loading the entire object. This can help to reduce the amount of data that is transferred between the application and the database, and can improve the performance of your application.
To load partial data using anonymous objects in XPO, you can create a LINQ query that selects only the properties that you need from the object. For example, suppose you have a Customer
object that has properties for FirstName
, LastName
, City
, State
, and ZipCode
. If you only need to load the FirstName
and LastName
properties, you can create a LINQ query like this:
var customers = from c in session.Query<Customer>() where c.City == "New York" select new { c.FirstName, c.LastName };
This will allow us to load only the data that we need from the database, rather than loading the entire Customer
object. Translation: faster query times and a more responsive application.
That’s a wrap for this article!
Remember:
If you are using DevExpress ORM XPO in your .NET application, the XPQuery class is a must-have tool that will help you to easily and efficiently query your data.
Until next time, XPO out!
#When you get the same questions 3 times you should write an article
#NotesToSelf