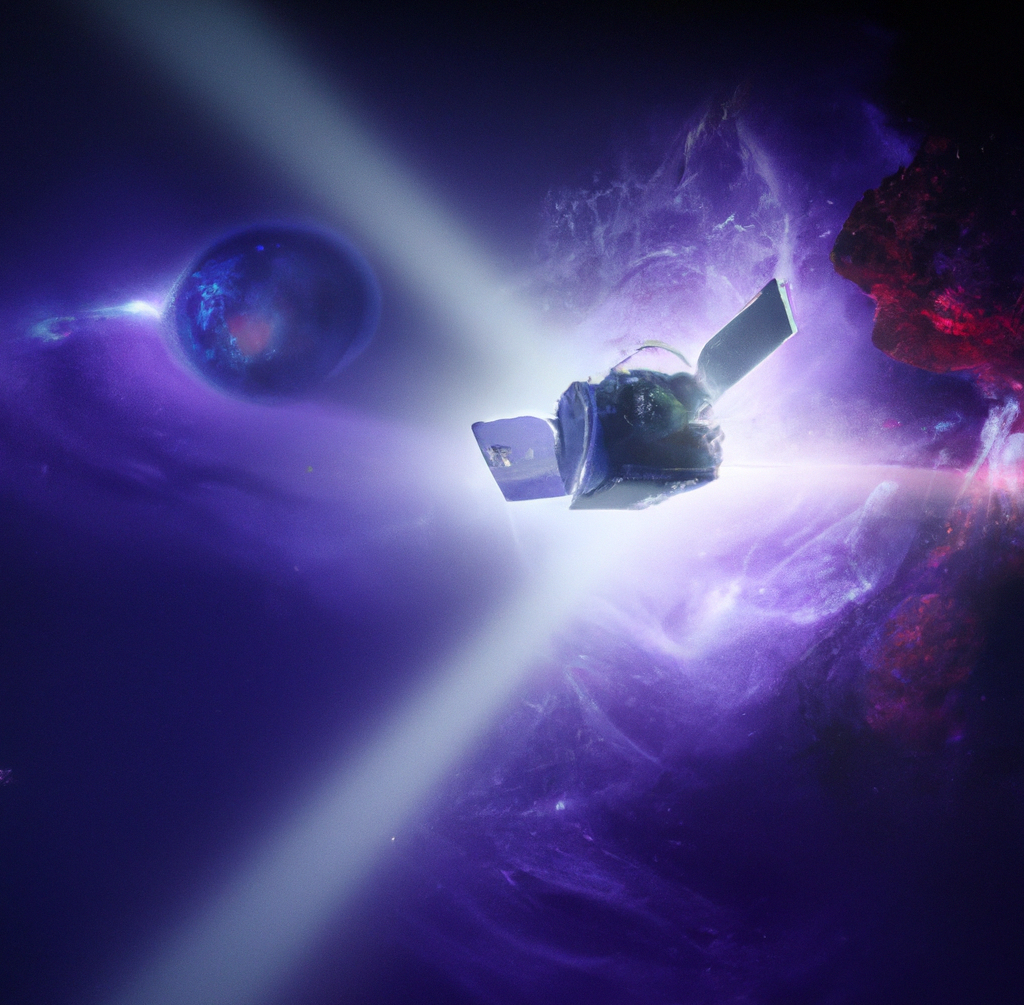
What is the ObjectSpace Class?
The ObjectSpace class in DevExpress XAF acts as a mediator between your application and the underlying data store. It serves as an abstraction layer that allows you to perform various data-related operations, such as creating, modifying, and deleting objects, without directly interacting with the data store or dealing with complex database operations.
The ObjectSpace class in DevExpress XAF is an implementation of the IObjectSpace interface, providing a unified set of methods for data manipulation. This interface includes essential methods such as CreateObject, GetObjectByKey, Delete, and CommitChanges, among others.
It’s important to note that the ObjectSpace class is ORM-agnostic, meaning it can work seamlessly with both Entity Framework Core (EF Core) and eXpress Persistent Objects (XPO).
DevExpress also provides built-in ObjectSpaces tailored for specific scenarios, such as XPObjectSpace for XPO, EFCoreObjectSpace for EF Core, and NonPersistentObjectSpace for non-persistent objects. Additionally, there are secured versions of these ObjectSpaces available, ensuring data integrity and access control in secure application environments.
How to Use the ObjectSpace?
To utilize the ObjectSpace class effectively, you must understand its lifecycle and how it interacts with XAF’s application model. Here are the key steps to using the ObjectSpace class:
- Creating A New ObjectSpace: You can obtain an instance of the ObjectSpace class within your XAF application using the XafApplication object. The ObjectSpace is also typically retrieved from the current View or Controller.
var objectSpace = Application.CreateObjectSpace(typeof(Order));
- Creating Objects: To create a new object using the ObjectSpace, you need to call the ObjectSpace’s CreateObject method to instantiate the required persistent object type and add it to the ObjectSpace. You can then set its properties and call the ObjectSpace’s CommitChanges method to save it to the data store.
var newOrder = objectSpace.CreateObject<Order>(); newOrder.OrderDate = DateTime.Now; newOrder.Customer = objectSpace.FindObject<Customer>(CriteriaOperator.Parse("Name = ?", "John Doe")); objectSpace.CommitChanges();
- Modifying Objects: To modify an existing object, you can retrieve it from the ObjectSpace, modify its properties, and again call the ObjectSpace’s CommitChanges method to save the changes.
var order = objectSpace.GetObjectByKey<Order>(orderId); order.TotalAmount += 100; objectSpace.CommitChanges();
- Deleting Objects: To delete an object, you can call the ObjectSpace’s Delete method with the object to be removed and then commit the changes.
var order = objectSpace.GetObjectByKey<Order>(orderId); objectSpace.Delete(order); objectSpace.CommitChanges();
- Handling Object Associations: The ObjectSpace class also allows you to manage object associations, such as setting relationships between objects or retrieving related objects.
var customer = objectSpace.GetObjectByKey<Customer>(customerId); var newOrder = objectSpace.CreateObject<Order>(); newOrder.Customer = customer; objectSpace.CommitChanges();
Sample Code: Let’s put it all together in a really simple code snippet:
var objectSpace = Application.CreateObjectSpace(typeof(Order)); var newOrder = objectSpace.CreateObject<Order>(); newOrder.OrderDate = DateTime.Now; newOrder.Customer = objectSpace.FindObject<Customer>(CriteriaOperator.Parse("Name = ?", "John Doe")); objectSpace.CommitChanges();
And that’s a wrap for this article!
We covered how the ObjectSpace class plays a vital role in managing data operations within DevExpress XAF applications. By utilizing the ObjectSpace class effectively, you can abstract away the complexities of data manipulation, focusing more on building robust business logic. Understanding the lifecycle and usage of the ObjectSpace class will empower you to create efficient and scalable applications using DevExpress XAF.
Update: One last thing related to XPO. We get the question about using the Save method vs the CommitChanges at least a couple times a month.
Here is the answer from the docs:
The Save method saves the object immediately when the current persistent object is loaded using Session. If the UnitOfWork is used, the method marks the persistent object as modified and forces the UnitOfWork to include it into the further commit operation. UnitOfWork automatically collects new objects and tracks modified objects using the OnChanged method.
Translation: In XAF we use the ObjectSpace’s CommitChanges method.
Until next time, XAF out!
#When you get the same questions 3 times you should write an article
#NotesToSelf